First, the TDD folks would say, “write tests.” If you’re not doing that, continue reading for an alternative.
Here’s the process I follow:
0. Isolate: Think about the parts of your app that might be causing trouble. What is it doing? What should it be doing? And where is the code that should be handling that? Above all, READ THE ERROR MESSAGE (if any). I find myself seeing the an error message then flipping back to my code. After looking at the code for a while, I generally go back to the error and see that something there is really helpful in pinpointing the problem. I could save those two minutes if I would reverse that process.
1. Diagnose: Find the bugs.
Methods –
a. Comment out parts of your code starting at the bottom of the broken block and test. Keep going until your program is working. Then, you’ll know where the offending code is (i.e., the piece of code you just commented out).
b. Use debug statements (print_r(), console.log(), etc.) to test out your variables. Today I was working with some jQuery. I had a selector like $('.div1').parent().attr('data-id');
For some reason, I wasn’t getting the value of 'data-id'
, just undefined
. So, I console.log’ed each piece of the selector and then the .attr()
method:
console.log($('.div1'));
console.log($('.div1').parent());
console.log($('.div1').parent().attr('data-id'));
The results showed me that I was selecting the wrong (a non-existent) DOM node, and I fixed the problem.
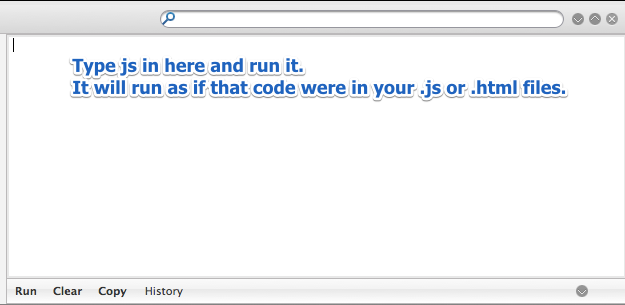
Also, use the JavaScript console in your browser to test your selectors and other code. Type your selector in the console and run it. If you see undefined in the console log to the left, your selector isn’t selecting a valid DOM node. Tweak it until you’ve got what you’re looking for.
2. Prescribe: You’ve found the problem. Now, before jumping to a solution (unless it’s obvious), think about/plan a solution. Code edit, refresh, code edit, refresh, code edit, refresh, repeat is a painful and confusing way to code. You may end up with more bugs than when you began. So think first.
3. Treat: Now that you know what to do, try out your corrections. If the first doesn’t work, go back
to #2 and think again.